Difficulty: Medium
Here we will cover how to disable payment gateways for certain countries on your checkout page in your Woo commerce store. We will use inspect element to get the id of this payment gateways and then we will use that id in our code to disable them.
Table of Contents
TLDR Section
*Don’t forget to replace <payment_gateway_id> and <Country_Code> in the code below.
**If you don’t know where to find this, just follow the guide (it takes 2 minutes) or look at our cheatsheet at the bottom of the page.
TLDR
File: Functions.php
add_filter( 'woocommerce_available_payment_gateways', 'payment_gateway_disable_country' );
function payment_gateway_disable_country( $available_gateways ) {
if ( is_admin() ) return $available_gateways;
if ( isset( $available_gateways['<payment_gateway_id>'] ) && WC()->customer->get_billing_country() == '<Country_Code>' ) {
unset( $available_gateways['<payment_gateway_id>'] );
}
return $available_gateways;
}
Finding Payment Gateway Id
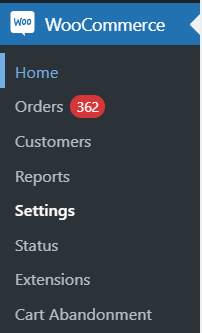
To find payment id we need to navigate to admin interface and then under WooCommerce select Settings.
After navigating there you will see a window like this one below. In there you will need to press right click on one of those payment gateways and select inspect element (on some browsers it just says inspect).
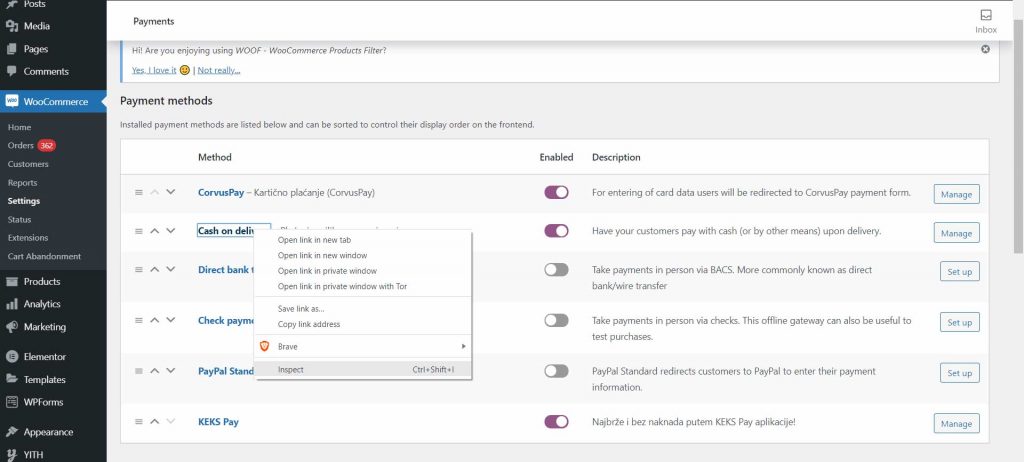
After clicking inspect element a windows like this one below will open and there we will find id for all of our payment methods. I suggest to write them down cause we will need them in our next step.
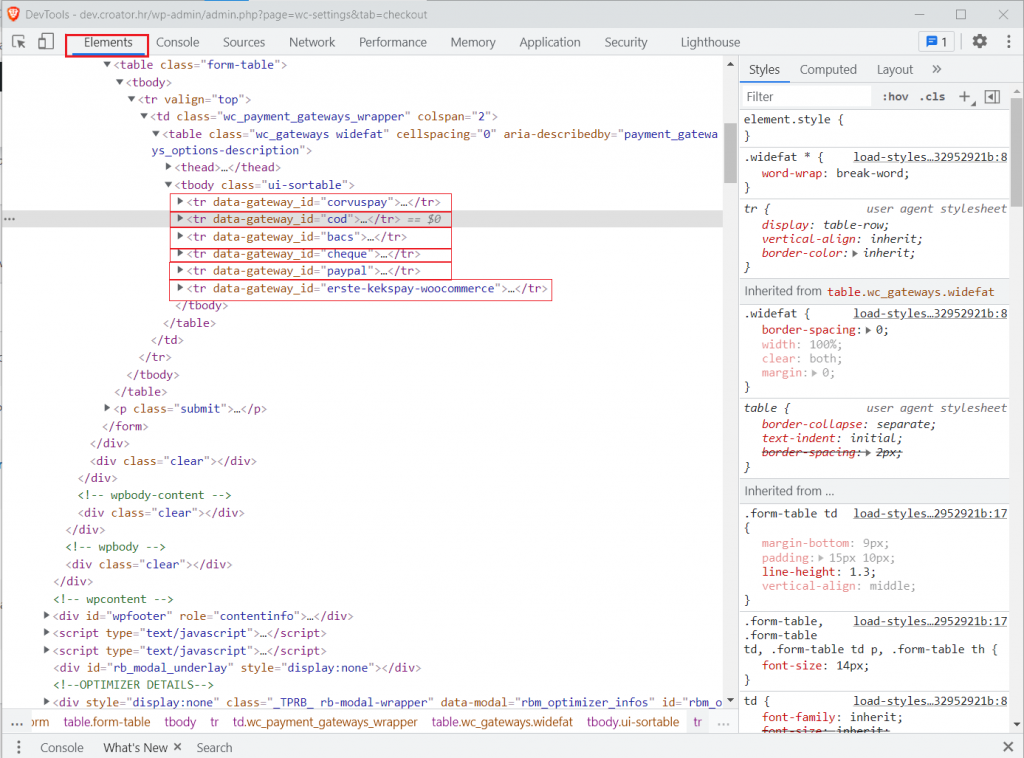
Disabling payment gateway for certain country
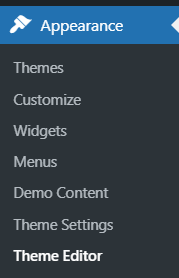
Okay, now that we got Payment ID we need to go to the admin interface and then go to Appearance and then under Appearance select Theme Editor
After navigating there you will see a windows like this one below. In there select Theme Functions (functions.php) and then at the end put this code.
*Don’t forget to replace <payment_gateway_id> with payment gateway id
**Don’t forget to replace <Country_Code> with country code
add_filter( 'woocommerce_available_payment_gateways', 'payment_gateway_disable_country' );
function payment_gateway_disable_country( $available_gateways ) {
if ( is_admin() ) return $available_gateways;
if ( isset( $available_gateways['<payment_gateway_id>'] ) && WC()->customer->get_billing_country() == '<Country_Code>' ) {
unset( $available_gateways['<payment_gateway_id>'] );
}
return $available_gateways;
}
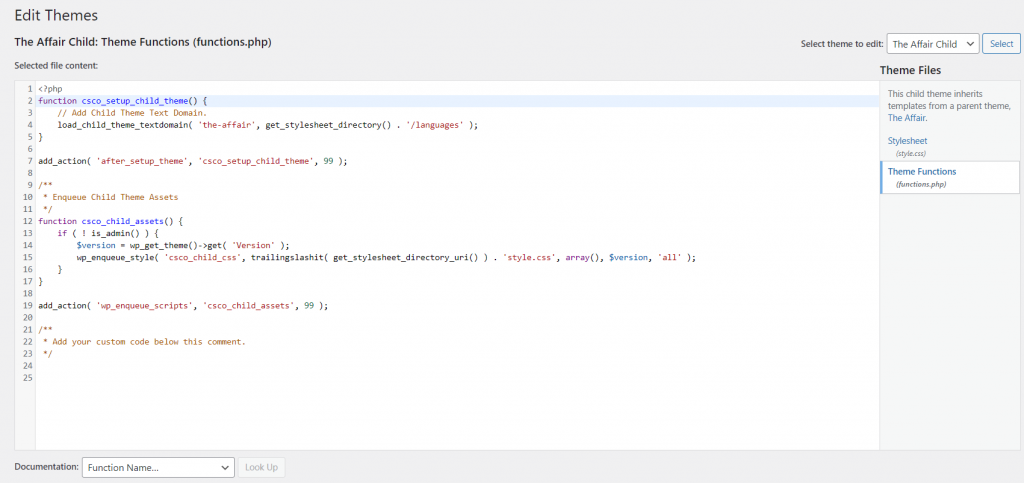
Editing the code
There isn’t much you can do with this one, except maybe blocking more than one payment gateway in certain country or blocking one payment gateway in multiple countries. To accomplish that you can just add to the code above else and if statement and put there another payment id and country code you want to block
add_filter( 'woocommerce_available_payment_gateways', 'payment_gateway_disable_country' );
function payment_gateway_disable_country( $available_gateways ) {
if ( is_admin() ) return $available_gateways;
if ( isset( $available_gateways['<payment_gateway_id>'] ) && WC()->customer->get_billing_country() == '<Country_Code>' ) {
unset( $available_gateways['<payment_gateway_id>'] );
} else {
if ( isset( $available_gateways['<payment__gateway_id>'] ) && WC()->customer->get_billing_country() == '<Country_Code>' ) {
unset( $available_gateways['<payment_gateway_id>'] );
}
}
return $available_gateways;
}
Payment gateway ID Cheatsheet
corvuspay – CorvusPay
cod – Cash on delivery
bacs – Direct bank transfer
cheque – Check payments
paypal – PayPal Standard
erste-kekspay-woocommerce -KEKS Pay