Difficulty: Easy
In this guide we will show you how to remove Woocommerce checkout fields. Guide will show you how to remove certain or all fields from your checkout page in your Woocommerce store. Removing checkout fields is very simple and can be done like many other things on your WooCommerce store by adding code to your Functions.php file in your theme folder.
TLDR SECTION
// Remove checkout fields
add_filter( 'woocommerce_checkout_fields' , 'woowiki_remove_checkout_fields' );
function woowiki_remove_checkout_fields( $fields ) {
// remove billing fields
// If you don't want to remove some of this fields just remove or comment out those line of code
unset($fields['billing']['billing_first_name']); // Unsets Billing First name
unset($fields['billing']['billing_last_name']); // Unsets Billing Last name
unset($fields['billing']['billing_company']); // Unsets Billing company
unset($fields['billing']['billing_address_1']); // Unsets Billing Address 1
unset($fields['billing']['billing_address_2']); // Unsets Billing Address 2
unset($fields['billing']['billing_city']); // Unsets Billing city
unset($fields['billing']['billing_postcode']); // Unsets Billing postcode
unset($fields['billing']['billing_country']); // Unsets Billing country
unset($fields['billing']['billing_state']); // Unsets Billing state
unset($fields['billing']['billing_phone']); // Unsets Billing phone
unset($fields['billing']['billing_email']); // Unsets Billing email
// remove shipping fields
unset($fields['shipping']['shipping_first_name']); // Unsets Shipping first name
unset($fields['shipping']['shipping_last_name']); // Unsets Shipping last name
unset($fields['shipping']['shipping_company']); // Unsets Shipping company
unset($fields['shipping']['shipping_address_1']); // Unsets Shipping address 1
unset($fields['shipping']['shipping_address_2']); // Unsets Shipping address 2
unset($fields['shipping']['shipping_city']); // Unsets Shipping city
unset($fields['shipping']['shipping_postcode']); // Unsets Shipping postcode
unset($fields['shipping']['shipping_country']); // Unsets Shipping country
unset($fields['shipping']['shipping_state']); // Unsets Shipping state
// remove order comment fields
unset($fields['order']['order_comments']); // Unsets Order comments
return $fields;
}
Removing Woocommerce checkout fields
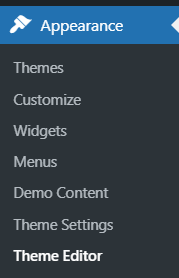
In order to remove some or all checkout fields from your checkout page we need to navigate to your admin interface and then go to Appearance and then under Appearance select Theme Editor.
After navigating there you will see a window like this one below. On the right side select Theme Functions (functions.php).
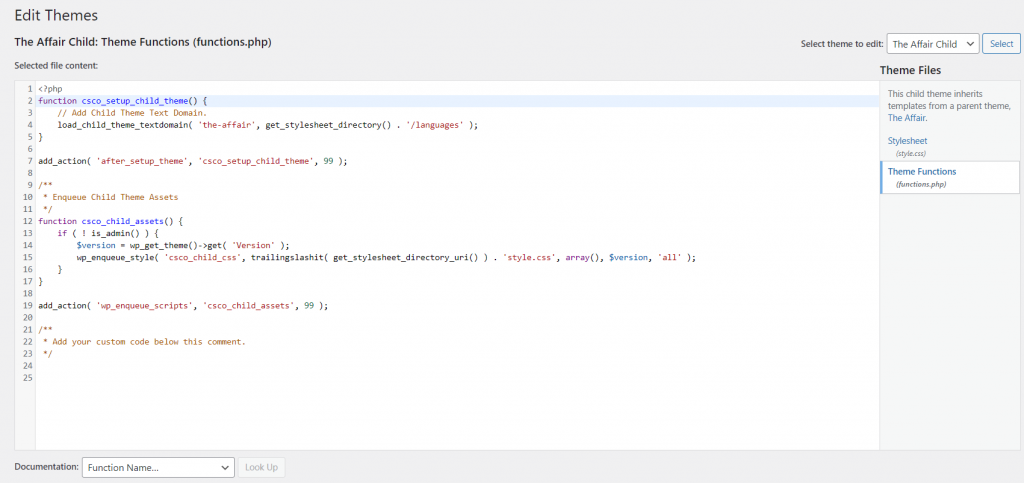
Copy-Paste the code provided below at the end of functions.php file. Provided code removes all of the checkout fields on woocommerce page. In order to remove just some of the fields you will need to delete the unnecessary lines of code. For example if you would like to keep the field “First Name” in your checkout page you would remove the line of code where it says:
“unset($fields[‘billing’][‘billing_first_name’]); // Billing First name“.
Same thing applies for every other field you want to keep.
// Remove checkout fields
add_filter( 'woocommerce_checkout_fields' , 'remove_checkout_fields' );
function remove_checkout_fields( $fields ) {
// remove billing fields
unset($fields['billing']['billing_first_name']); // Unsets Billing First name
unset($fields['billing']['billing_last_name']); // Unsets Billing Last name
unset($fields['billing']['billing_company']); // Unsets Billing company
unset($fields['billing']['billing_address_1']); // Unsets Billing Address 1
unset($fields['billing']['billing_address_2']); // Unsets Billing Address 2
unset($fields['billing']['billing_city']); // Unsets Billing city
unset($fields['billing']['billing_postcode']); // Unsets Billing postcode
unset($fields['billing']['billing_country']); // Unsets Billing country
unset($fields['billing']['billing_state']); // Unsets Billing state
unset($fields['billing']['billing_phone']); // Unsets Billing phone
unset($fields['billing']['billing_email']); // Unsets Billing email
// remove shipping fields
unset($fields['shipping']['shipping_first_name']); // Unsets Shipping first name
unset($fields['shipping']['shipping_last_name']); // Unsets Shipping last name
unset($fields['shipping']['shipping_company']); // Unsets Shipping company
unset($fields['shipping']['shipping_address_1']); // Unsets Shipping address 1
unset($fields['shipping']['shipping_address_2']); // Unsets Shipping address 2
unset($fields['shipping']['shipping_city']); // Unsets Shipping city
unset($fields['shipping']['shipping_postcode']); // Unsets Shipping postcode
unset($fields['shipping']['shipping_country']); // Unsets Shipping country
unset($fields['shipping']['shipping_state']); // Unsets Shipping state
// remove order comment fields
unset($fields['order']['order_comments']); // Unsets Order comments
return $fields;
}
After you finished implementing the code. You need to click on button bellow to update your file/save changes and that’s it.
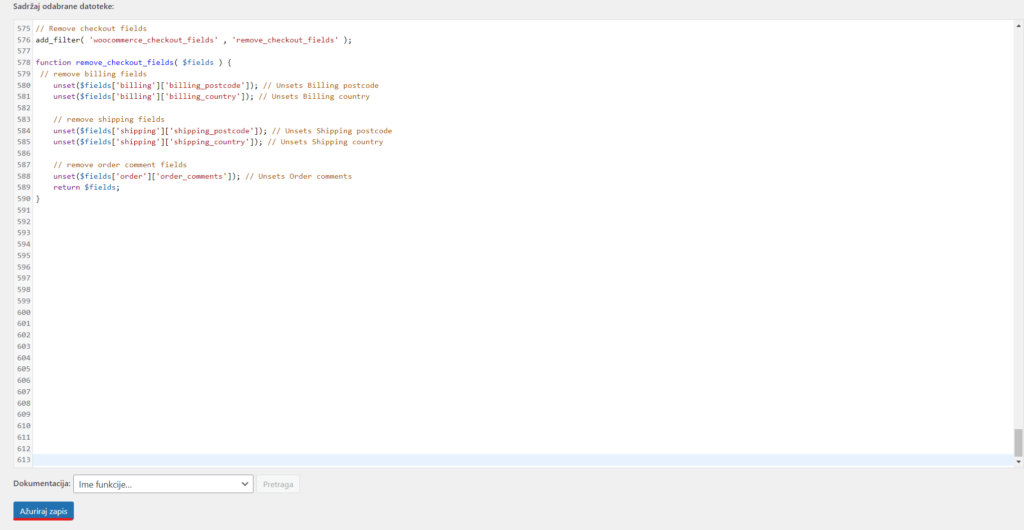
Now your WooCommerce checkout page will have the fields you desire.